mirror of
https://github.com/zsh-users/zsh-autosuggestions.git
synced 2025-04-17 11:35:31 +08:00
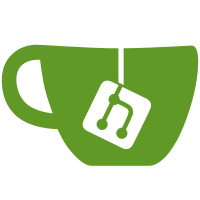
This strategy relies on the history being exactly in the order in which commands have been entered. Therefore, options like suppressing duplicates or expiring duplicates first will lead to unexpected suggestions.
53 lines
1.7 KiB
Bash
53 lines
1.7 KiB
Bash
|
|
#--------------------------------------------------------------------#
|
|
# Match Previous Command Suggestion Strategy #
|
|
#--------------------------------------------------------------------#
|
|
# Suggests the most recent history item that matches the given
|
|
# prefix and whose preceding history item also matches the most
|
|
# recently executed command.
|
|
#
|
|
# For example, suppose your history has the following entries:
|
|
# - pwd
|
|
# - ls foo
|
|
# - ls bar
|
|
# - pwd
|
|
#
|
|
# Given the history list above, when you type 'ls', the suggestion
|
|
# will be 'ls foo' rather than 'ls bar' because your most recently
|
|
# executed command (pwd) was previously followed by 'ls foo'.
|
|
#
|
|
# Note that this strategy won't work as expected with ZSH options that don't
|
|
# preserve the history order such as `HIST_IGNORE_ALL_DUPS` or
|
|
# `HIST_EXPIRE_DUPS_FIRST`.
|
|
|
|
_zsh_autosuggest_strategy_match_prev_cmd() {
|
|
local prefix="$1"
|
|
|
|
# Get all history event numbers that correspond to history
|
|
# entries that match pattern $prefix*
|
|
local history_match_keys
|
|
history_match_keys=(${(k)history[(R)$prefix*]})
|
|
|
|
# By default we use the first history number (most recent history entry)
|
|
local histkey="${history_match_keys[1]}"
|
|
|
|
# Get the previously executed command
|
|
local prev_cmd="$(_zsh_autosuggest_escape_command "${history[$((HISTCMD-1))]}")"
|
|
|
|
# Iterate up to the first 200 history event numbers that match $prefix
|
|
for key in "${(@)history_match_keys[1,200]}"; do
|
|
# Stop if we ran out of history
|
|
[[ $key -gt 1 ]] || break
|
|
|
|
# See if the history entry preceding the suggestion matches the
|
|
# previous command, and use it if it does
|
|
if [[ "${history[$((key - 1))]}" == "$prev_cmd" ]]; then
|
|
histkey="$key"
|
|
break
|
|
fi
|
|
done
|
|
|
|
# Echo the matched history entry
|
|
echo -E "$history[$histkey]"
|
|
}
|